TimeOfDay is one of Flutter classes but not from dart. It stores only hours and minutes. It doesn’t have year, month, date, seconds, or milliseconds. Why is it necessary in the first place?
If we want to pick a date and time, showDatePicker
and showTimePicker
functions are the first choice for the implementation. showTimePicker
returns TimeOfDay
and we need to handle it.
Current time
If we want to create an instance with the current time, we can use either now()
method or fromDateTime(datetime)
.
TimeOfDay.now().format(context);
TimeOfDay.fromDateTime(DateTime.now()).format(context);
toString
Unfortunately, toString returns the following result that doesn’t match our expectations.
final time = TimeOfDay(hour: 21, minute: 12);
time.toString(); // TimeOfDay(21:12)
We expect that it returns hh:mm format but actually not. I’m wondering who wants to use it.
format
TimeOfDay has format
method that converts to 12 hours format.
time.format(context); // 9:12 PM
There are many cases that 24 hours format is the more desired format.
Convert it to 24 hours format
Firstly, let’s check the format implementation.
String format(BuildContext context) {
assert(debugCheckHasMediaQuery(context));
assert(debugCheckHasMaterialLocalizations(context));
final MaterialLocalizations localizations = MaterialLocalizations.of(context);
return localizations.formatTimeOfDay(
this,
alwaysUse24HourFormat: MediaQuery.of(context).alwaysUse24HourFormat,
);
}
If we set alwaysUse24HourFormat
to true, it might convert to 24 hours format but we need to clone the default setting and then change the value somewhere. It seems to have more steps than the next solution.
Is the following the simplest way?
Text("24h: ${time.hour}:${time.minute}"); // 21:12
But if the number has only one digit, the result becomes an unexpected value.
final time = TimeOfDay(hour: 9, minute: 5);
Text("24h: ${time.hour}:${time.minute}"); // 9:5
Defining an extension for TimeOfDay is the simplest and a powerful way.
extension TimeOfDayConverter on TimeOfDay {
String to24hours() {
final hour = this.hour.toString().padLeft(2, "0");
final min = this.minute.toString().padLeft(2, "0");
return "$hour:$min";
}
}
Text("24h: ${time.to24hours()}"); // 09:05
Once we create this class, we can use it everywhere.
There are other properties as well. I took an image for you.
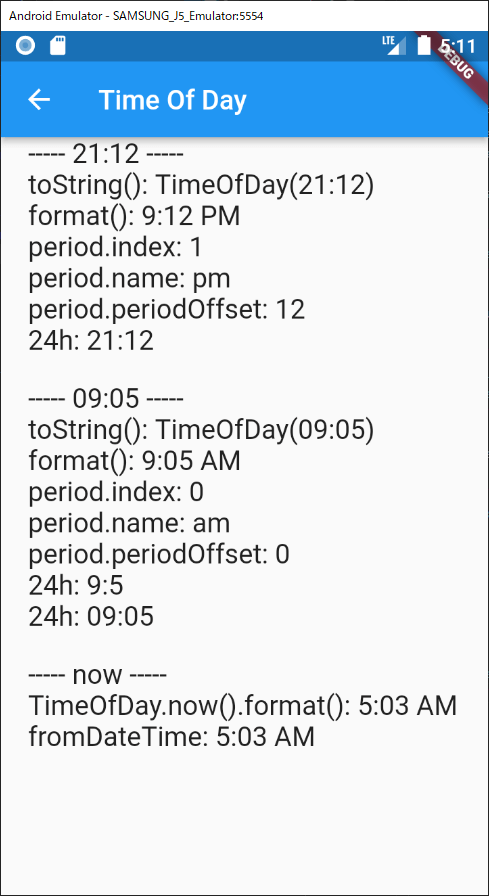
If you want to read the whole code, you can find it here.
Comments