Dropdown is one of the common ways to let a user select an option from a list. Let’s learn how to implement it in Flutter in this article.
My Flutter version is as follows.
$ flutter --version
Flutter 2.5.0 • channel stable • https://github.com/flutter/flutter.git
Framework • revision 4cc385b4b8 (8 weeks ago) • 2021-09-07 23:01:49 -0700
Engine • revision f0826da7ef
Tools • Dart 2.14.0
Set only required property
Firstly, let’s check the default behavior by assigning only the required parameter. The required parameter is only items property that requires List<DropdownMenuItem<T>>?
. We will use the following list and a function to create a DropdownMenuItem list.
final list = ["Apple", "Orange", "Kiwi", "Banana", "Grape"];
List<DropdownMenuItem<String>> _createList() {
return list
.map<DropdownMenuItem<String>>(
(e) => DropdownMenuItem(
value: e,
child: Text(e),
),
)
.toList();
}
final dropdown = DropdownButton(items: _createList());
The top dropdown is for this implementation.
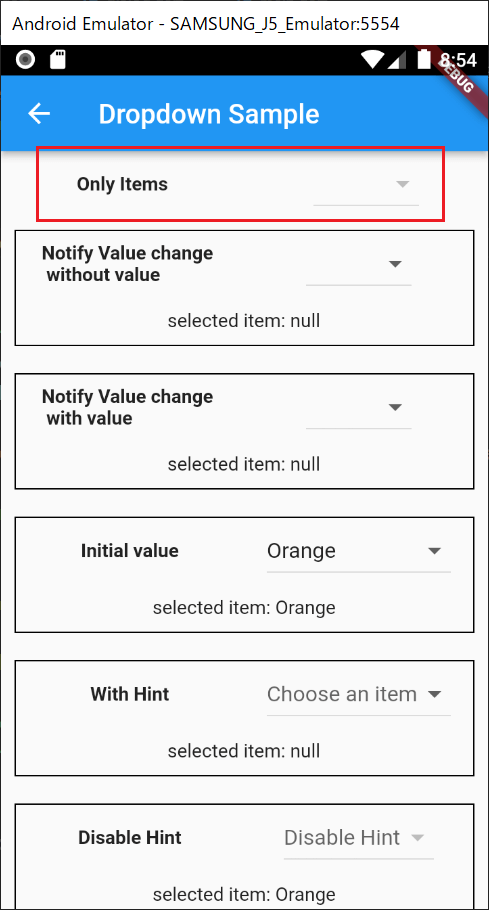
It shows an empty string and a user can’t change the value because it is disabled.
Notify value change / button enabled
We need to enable the dropdown button. For that, onChanged property needs to be set. Let’s assign the value to another variable to show the result.
String? _selectedItem1;
...
final dropdown = DropdownButton(
items: _createList(),
onChanged: (String? value) => setState(() {
_selectedItem1 = value ?? "";
}),
);
The selected item is null at first but its text changes when selecting an item from the dropdown menu. However, the dropdown still shows an empty string on it because the value property is not set.
Apply the change to the dropdown text
To change the dropdown text, we need to set the value property. Let’s prepare the variable.
String? _selectedItem2;
...
final dropdown = DropdownButton(
items: _createList(),
value: _selectedItem2,
onChanged: (String? value) => setState(() {
_selectedItem2 = value ?? "";
}),
);
The dropdown shows an empty string at first because it is null at first but the text on the dropdown changes when selecting an item from the list.
Set hint text instead of initial value
If we want to show hint text on the dropdown menu, the hint property is for it. This is useful if we don’t want to set an initial value but tell a user something like “choose an item” from the menu.
String? _selectedItem3;
...
final dropdown = DropdownButton(
items: _createList(),
hint: Text("Choose an item"),
value: _selectedItem3,
onChanged: (String? value) => setState(() {
_selectedItem3 = value ?? "";
}),
);
Set initial value
If we want to set an initial value to the dropdown, simply set the value to the value property. Its value must be included in the items list, otherwise, an error occurs.
String _selectedItem4 = "Orange";
...
final dropdown = DropdownButton(
items: _createList(),
hint: Text("Choose an item"),
value: _selectedItem4,
onChanged: (String? value) => setState(() {
_selectedItem4 = value ?? "";
}),
);
Show hint if the dropdown is disabled
If value and onChanged are null, the widget specified at disabledHint is shown.
final dropdown = DropdownButton(
items: _createList(),
disabledHint: Text("Disable Hint"),
);
Disable the dropdown
There are some cases that we want to disable the dropdown according to some conditions. Let’s add a button to enable/disable the dropdown.
bool _enable = true;
String _buttonText = "disable";
...
final disableButton = ElevatedButton(
onPressed: () => setState(() {
if (_enable) {
_enable = false;
_buttonText = "enable";
} else {
_enable = true;
_buttonText = "disable";
}
}),
child: Text(_buttonText),
);
The button text changes according to the dropdown state. As I mentioned above, we need to set null to onChanged property if we want to disable the dropdown.
String? _selectedItem5;
...
final onChanged = (value) => setState(() {
_selectedItem5 = value ?? "";
});
final dropdown = DropdownButton(
items: _createList(),
disabledHint: Text("Disable Hint"),
onChanged: _enable ? onChanged : null,
);
onChanged property is set only when the _enable is true.
disableHint is shown if the value is null and the state is disabled. Once a user selects an item from the list, the selected value is shown on the dropdown even if the state is disabled.
change dropdown menu color
If we want to change the color of the dropdown menu, set desired color to the dropdownColor property.
final backColor = DropdownButton(
items: _createList(),
onChanged: (value) {},
dropdownColor: Colors.cyan,
);

If you want to change the color of each item text, set the color in DropdownMenuItem.
DropdownMenuItem(
value: value,
child: Text(
value,
style: TextStyle(
fontWeight: FontWeight.bold,
color: Colors.red,
),
),
);

End
Go to my repository if you want to check the complete code.
I guess SimpleDialog can be used for the same purpose. Check this post if you want to use it.
Comments