I faced RenderFlex overflowed error while I was implementing Flutter app. I could eventually solve the problem by using ListView but I will show my case and what I did before using ListView.
Overflowed error happens only when keyboard appears
I wrote the following code.
return Scaffold(
appBar: AppBar(
title: Text(widget.title),
),
body:
Padding(
padding: EdgeInsets.all(10),
child: Column(
crossAxisAlignment: CrossAxisAlignment.start,
children: [
Padding(child: nameField, padding: EdgeInsets.only(bottom: 20)),
Padding(child: typeRow, padding: EdgeInsets.only(bottom: 20)),
Padding(child: unitRow, padding: EdgeInsets.only(bottom: 20)),
Padding(child: categoryRow, padding: EdgeInsets.only(bottom: 20)),
],
),
)
);
It looks like this.
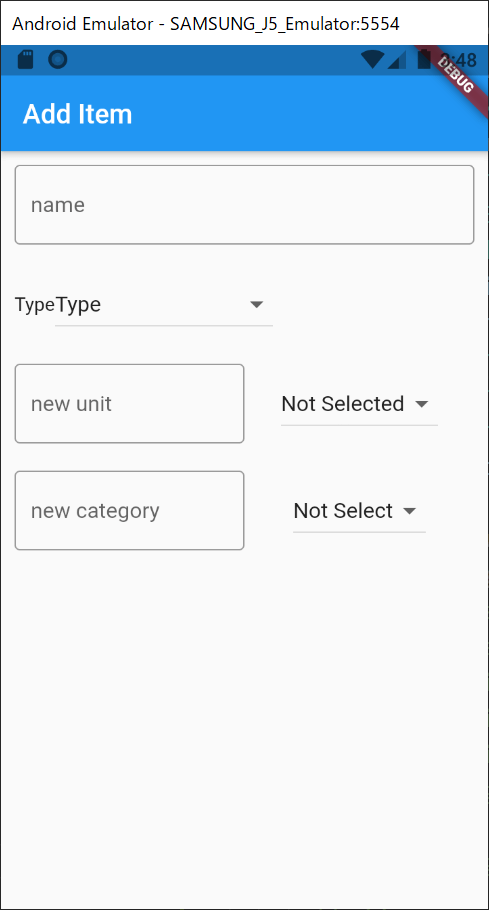
When it starts up error doesn’t occur but it occurs when I focus on text field.
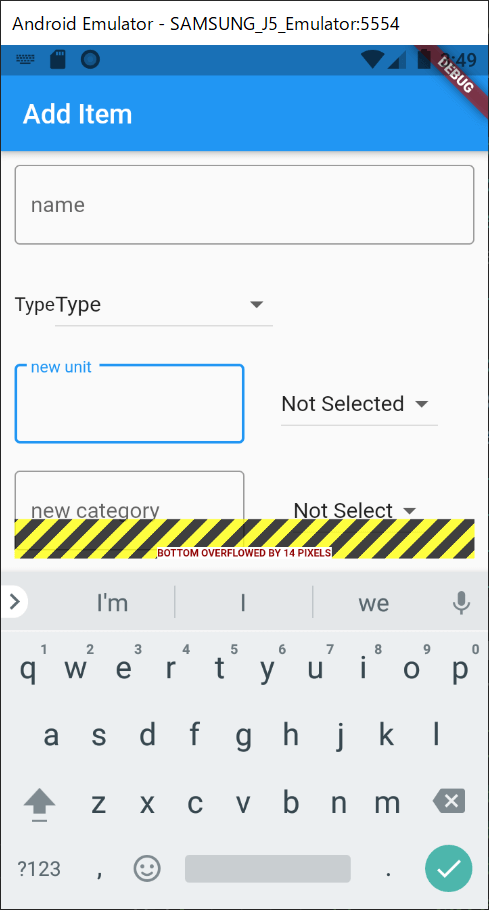
I didn’t understand at first why it happened only when the keyboard appeared. It’s because the keyboard occupies almost half of the screen and the size of the body for the Scaffold decreases. Therefore, it leads to an overflow error. The message I got was the following.
════════ Exception caught by rendering library ═════════════════════════════════ The following assertion was thrown during layout: A RenderFlex overflowed by 14 pixels on the bottom. The relevant error-causing widget was Column lib\AddItemView.dart:109 You can inspect this widget using the 'Inspect Widget' button in the VS Code notification. The overflowing RenderFlex has an orientation of Axis.vertical. The edge of the RenderFlex that is overflowing has been marked in the rendering with a yellow and black striped pattern. This is usually caused by the contents being too big for the RenderFlex. Consider applying a flex factor (e.g. using an Expanded widget) to force the children of the RenderFlex to fit within the available space instead of being sized to their natural size. This is considered an error condition because it indicates that there is content that cannot be seen. If the content is legitimately bigger than the available space, consider clipping it with a ClipRect widget before putting it in the flex, or using a scrollable container rather than a Flex, like a ListView. The specific RenderFlex in question is: RenderFlex#54ac3 relayoutBoundary=up2 OVERFLOWING
What I tried first was, as the message described, to use Expanded widget for Column. The official page describes the same thing.
Well, you need to make sure the Column won’t attempt to be wider than it can be. To achieve this, you need to constrain its width. One way to do it is to wrap the Column in an Expanded widget:
https://flutter.dev/docs/testing/common-errors#a-renderflex-overflowed
I added Expanded widget.
return Scaffold(
appBar: AppBar(
title: Text(widget.title),
),
body: Padding(
padding: EdgeInsets.all(10),
child: Expanded( // Expanded is added
child: Column(
crossAxisAlignment: CrossAxisAlignment.start,
children: [
Padding(child: nameField, padding: EdgeInsets.only(bottom: 20)),
Padding(child: typeRow, padding: EdgeInsets.only(bottom: 20)),
Padding(child: unitRow, padding: EdgeInsets.only(bottom: 20)),
Padding(
child: categoryRow, padding: EdgeInsets.only(bottom: 20)),
],
),
),
),
);
However, the result was the same.
ListView fixes the overflow error
When the keyboard appears the screen size for the app decreases. Therefore we need to make the view scrollable. Otherwise, some widgets can be hidden under the keyboard which leads RenderFlex overflowed error.
The final code that I could solve the problem is the following.
return Scaffold(
appBar: AppBar(
title: Text(widget.title),
),
body: ListView(
children: [
Padding(child: nameField, padding: EdgeInsets.only(bottom: 20)),
Padding(child: typeRow, padding: EdgeInsets.only(bottom: 20)),
Padding(child: unitRow, padding: EdgeInsets.only(bottom: 20)),
categoryRow,
],
padding: EdgeInsets.all(10),
),
);
The error message also says that ListView is another solution.
consider clipping it with a ClipRect widget before putting it in the flex, or using a scrollable container rather than a Flex, like a ListView.
End
I’m new to Flutter but I like it so far because UI can be written in code. The code will easily be messy because we need to wrap a widget by widget to set style for example. That’s an issue that I should take care of.
Comments