If text style needs to be applied to a Text widget, it’s easy to apply but if there are many widgets we don’t want to add a property to all widgets. Isn’t there a good way to apply the same text style to a certain widget tree?
Yes, there is. Let’s have a look at the solution.
Undesired implementation
If we don’t know how to apply the same text style to a group, we need to add the style to all widgets in the following way.
class _MyTextStyle {
static final style1 = TextStyle(
fontSize: 40,
color: Colors.red,
fontStyle: FontStyle.italic,
);
}
Text("AAA", style: _MyTextStyle.style1),
Text("BBB", style: _MyTextStyle.style1),
Text("CCC", style: _MyTextStyle.style1),
The style is set to the style property in all widgets. It’s cumbersome.
Change the default style by DefaultTextStyle.merge
We don’t want to set style property to all widgets but we want to set our own text style. It means that we want to set the default text style. DefaultTextStyle.merge
offers the feature. The usage is straightforward.
DefaultTextStyle.merge(
style: _MyTextStyle.style1,
child: _children(),
),
The specified style is applied to the whole widget tree. Let’s compare the two, that’s with/without DefaultTextStyle.merge
.
SingleChildScrollView(
child: Column(
children: [
_children(),
SizedBox(height: 20),
DefaultTextStyle.merge(
style: _MyTextStyle.style1,
child: _children(),
),
],
),
),
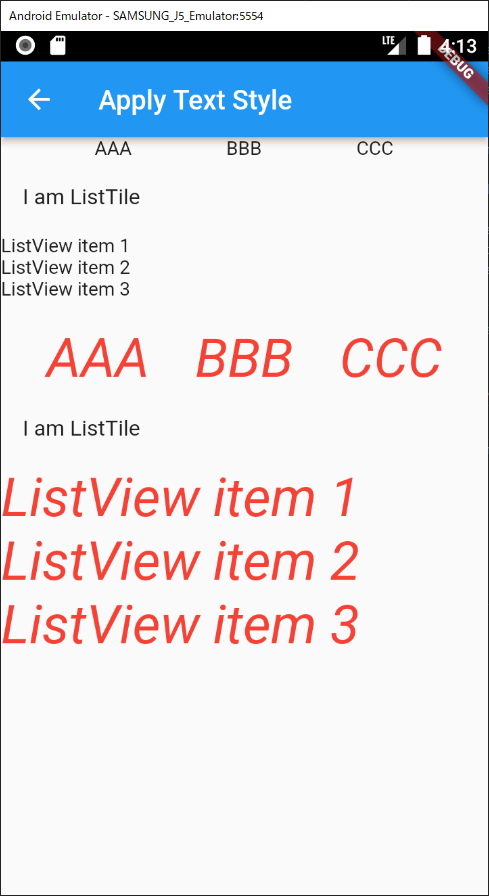
The same text style is applied to all normal text widgets!
Buuuuuuut
The style is NOT applied to a ListTile. There are probably some widgets that the specified text style isn’t applied like this. I found the following implementation in list_tile.dart file. Keep in mind this.
final Widget titleText = AnimatedDefaultTextStyle(
style: titleStyle,
duration: kThemeChangeDuration,
child: title ?? const SizedBox(),
);
Whole code
import 'package:flutter/material.dart';
class _MyTextStyle {
static final style1 = TextStyle(
fontSize: 40,
color: Colors.red,
fontStyle: FontStyle.italic,
);
}
class ApplyTextStyle extends StatelessWidget {
@override
Widget build(BuildContext context) {
return SafeArea(
child: Scaffold(
appBar: AppBar(
title: Text("Apply Text Style"),
),
body: SingleChildScrollView(
child: Column(
children: [
_children(),
SizedBox(height: 20),
DefaultTextStyle.merge(
style: _MyTextStyle.style1,
child: _children(),
),
],
),
),
),
);
}
Widget _children() {
return Column(
children: [
Row(
mainAxisAlignment: MainAxisAlignment.spaceEvenly,
children: [
Text("AAA"),
Text("BBB"),
Text("CCC"),
],
),
ListTile(title: Text("I am ListTile")),
ListView(
shrinkWrap: true,
children: [
Text("ListView item 1"),
Text("ListView item 2"),
Text("ListView item 3"),
],
),
],
);
}
}
Comments