I saw triple quotes in a class definition like the following.
class MyClass():
"""
This is my class.
It does something for you.
"""
...
It looks like a comment for the class. I didn’t know what it was.
Triple quotes can be used for a multi-line string and docstring. Let’s learn the usage here.
Triple quotes for multi-line string
When we want to write a multi-line string, we can write it in the following way.
print("Hello\nHow are you?\nI'm Yuto.\n")
# Hello
# How are you?
# I'm Yuto.
The sentence within the double quotes is handled as string type. \n
adds a new line.
But if it’s a long sentence, it becomes unreadable. We want to somehow make it clearer.
print("Hello\n"
"How are you?\n"
"I'm Yuto.\n")
This is more readable than before but if the sentence is long, it’s tedious to add a new line code \n
and double quotes.
In this case, we can use triple quotes here.
print("""Hello
How are you?
I'm Yuto.
""")
The code above shows the same result as before but if you write in the following way, the output becomes different.
print("""
Hello
How are you?
I'm Yuto.
""")
#
# Hello
# How are you?
# I'm Yuto.
The first line starts with a new line. Therefore it puts a new line to the output.
By the way, three single quotes can also work.
print('''Hello
How are you?
I'm Yuto.
''')
A variable can be used by prepending f
and use the variable with curly brackets.
additional = "additional information here"
print(f"""
Hello
How are you?
I'm Yuto.
{additional}
""")
# Hello
# How are you?
# I'm Yuto.
# additional information here
Triple quotes for docstrings
Triple quotes can also be used when we want to add a comment to a function or class. It’s essential to write such a comment to make it clear. Especially when we provide a module to other users.
Docstrings for a module
If we want to add a comment to the whole module, the comment must be written at the top of the file.
# triple_quotes.py
"""triple_quotes examples. module comment here """
print("Hello\nHow are you?\nI'm Yuto.\n")
...
When it’s imported in another file, the comment is shown like this below.

Docstrings for a function
Let’s see how to add docstrings to a function.
def doSomething():
"""
documentation here
first line
second line here
"""
print("Hello")
A new line doesn’t break the line. If the sentence needs to be shown on the new line, the sentence needs to be written after an empty line break.
The comment is shown when writing the function name.
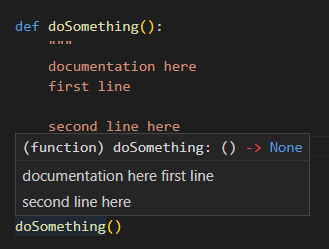
The comment can be written on a single line too.
def doSomething():
""" comment can be written on a single line"""
print("Hello")
But the comment must be written inside of the function. It doesn’t work properly if the comment is written in the following position.
# NOT WORK
""" something """
def notWork():
print("a")
"""something"""
return 3
Docstrings for a variable
Docstrings can also be used for a variable.
str_val = "This is a string value"
""" It contains important information"""
Likewise, add the comment within the triple quotes below the variable name.
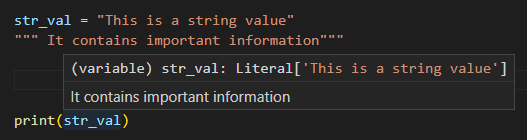
Docstrings for a class
You already know where to add the comment. A class has variables and functions. So, let’s add the docstrings.
class MyClass():
"""
This is my class.
Here it should summarize this class behavior
"""
x = 1
""" This is a member"""
def __init__(self, x):
""" This is constructor information."""
self.x = x
pass
def doSomething(self):
""" Print do something to the console """
print("do something")
def sayHello(self):
""" This function writes "Hello" to the console """
print("Hello")
self.doSomething()
All info except for the comment under class name is shown.
The class info is not shown on the constructor call. Instead, the info for __init__
function is shown because it is a constructor. If there is no docstring defined for the constructor, IntelliSense shows the class info like this below.

Docstring Format types
There are several format types of Docstrings. Learning one of them and using the same format in a project is better for the readability.
Google Python Style Guide
Example Google Style Python Docstrings
Comments