The main purpose of this article is to understand how the following code works. This is one of the ways to add element(s) to the list.
data = [1, 2, 3, 4]
data[:0] = [-1, -2, 0]
But it’s the first time for me to use list. Let’s learn other ways too.
Add an element to the first
We can simply add an element to the first place in this way.
data = [1, 2, 3, 4]
data.insert(0, 0)
print(data) # [0, 1, 2, 3, 4]
But if the added element is a list, it is added as a list.
data = [1, 2, 3, 4]
data.insert(0, [-2, -1])
print(data) # [[-2, -1], 1, 2, 3, 4]
Using square brackets for a list
If we want to add a list to the existing list, we can write in the following way.
data = [1, 2, 3, 4]
data[:0] = [-2, -1]
print(data) # [-2, -1, 1, 2, 3, 4]
Here, we need to know how the brackets with colon works [:0]
.
What if we put 0 on the left side?
data = [1, 2, 3, 4]
data[0:] = [-2, -1]
print(data) # [-2, -1]
Oh, all elements have gone.
Why?
What does square brackets with colon return
The syntax returns something. Let’s clarify it first.
data = [1, 2, 3, 4]
print(data[0]) # 1
print(data[0:]) # [1, 2, 3, 4]
print(data[2:]) # [3, 4]
print(data[3:]) # [4]
print(data[-1:]) # [4]
print(data[-2:]) # [3, 4]
This syntax returns all the elements from the specified index. If the number is negative, it is counted from the end. The index is used for the place but I think it’s easier to understand how it works if we give the index to the comma. [2:] returns all elements placed to the right side of the second index
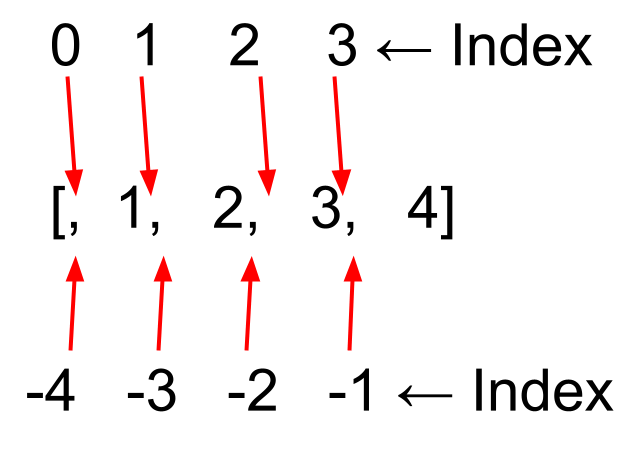
Let’s check the behavior when specifying the value on the right side only.
data = [1, 2, 3, 4]
print(data[:0]) # []
print(data[:2]) # [1, 2]
print(data[:3]) # [1, 2, 3]
print(data[:-1]) # [1, 2, 3]
print(data[:-2]) # [1, 2]
The result looks opposite to the previous result. This syntax returns all the elements between index 0 to the specified index.
From these two experiments, we can imagine how it works when a value is specified to both sides.
data = [1, 2, 3, 4, 5, 6, 7, 8, 9]
print(data[3: 7]) # [4, 5, 6, 7]
Adding elements to the first by using square brackets
Let’s go back to the original question described at the top of this article.
data = [1, 2, 3, 4]
data[:0] = [-1, -2, 0]
print(data) # [-1, -2, 0, 1, 2, 3, 4]
What if we change the index?
data = [1, 2, 3, 4]
data[:2] = [-1, -2, 0]
print(data) # [-1, -2, 0, 3, 4]
The first two entries were replaced with new entries.
Adding elements to the end
If we want to add an element to the end, we can use append
function.
data = [1, 2, 3, 4]
data.append(5)
print(data) # [1, 2, 3, 4, 5]
But it appends only one element. Thus, when the element is a list, the result becomes like this.
data = [1, 2, 3, 4]
data.append([5, 6])
print(data) # [1, 2, 3, 4, [5, 6]]
If we use the insert function, we can write it in this way.
data = [1, 2, 3, 4]
data.insert(len(data), 5)
print(data) # [1, 2, 3, 4, 5]
data = [1, 2, 3, 4]
data.insert(len(data), [5, 6])
print(data) # [1, 2, 3, 4, [5, 6]]
But if the added list needs to be expanded, extend
function can be used.
data = [1, 2, 3, 4]
data.extend([5, 6])
print(data) # [1, 2, 3, 4, 5, 6]
Adding elements to the end by using square brackets
I thought it was also possible to use square brackets when adding elements to the end.
But the result was different from what I expected.
To add elements to the front, we can add them in this way as has already been shown above.
data = [1, 2, 3, 4]
data[:0] = [-1, -2, 0]
print(data) # [-1, -2, 0, 1, 2, 3, 4]
data = [1, 2, 3, 4]
data[:2] = [-1, -2, 0]
print(data) # [-1, -2, 0, 3, 4]
What if we specify the value on the left side? Let’s try it.
data = [1, 2, 3, 4]
print(data[0:]) # [1, 2, 3, 4]
data[0:] = [-1, -2, 0]
print(data) # [-1, -2, 0]
data = [1, 2, 3, 4]
print(data[2:]) # [3, 4]
data[2:] = [-1, -2, 0]
print(data) # [1, 2, -1, -2, 0]
The returned elements are replaced with new elements.
Adding elements inbetween
It seems that the returned values are replaced with the new elements.
Then, what happens if we specify the value on both sides?
data = [1, 2, 3, 4, 5, 6, 7]
print(data[1:2]) # [2]
data[1:2] = [99, 100]
print(data) # [1, 99, 100, 3, 4, 5, 6, 7]
data = [1, 2, 3, 4, 5, 6, 7]
print(data[1:6]) # [2, 3, 4, 5, 6]
data[1:6] = [99, 100]
print(data) # [1, 99, 100, 7]
Yes, the returned values are replaced here as well.
Overview
The returned values are replaced with new values.
# --- New elements to the front ---
data = [1, 2, 3, 4]
print(data[:0]) # []
data[:0] = [-2, -1]
print(data) # [-2, -1, 1, 2, 3, 4]
data = [1, 2, 3, 4]
print(data[:2]) # [1, 2]
data[:2] = [-1, -2, 0]
print(data) # [-1, -2, 0, 3, 4]
# --- New elements to the end ---
data = [1, 2, 3, 4]
print(data[0:]) # [1, 2, 3, 4]
data[0:] = [-1, -2, 0]
print(data) # [-1, -2, 0]
data = [1, 2, 3, 4]
print(data[2:]) # [3, 4]
data[2:] = [-1, -2, 0]
print(data) # [1, 2, -1, -2, 0]
# --- New elements inbetween ---
data = [1, 2, 3, 4, 5, 6, 7]
print(data[1:2]) # [2]
data[1:2] = [99, 100]
print(data) # [1, 99, 100, 3, 4, 5, 6, 7]
data = [1, 2, 3, 4, 5, 6, 7]
print(data[1:6]) # [2, 3, 4, 5, 6]
data[1:6] = [99, 100]
print(data) # [1, 99, 100, 7]
Comments